Describe the bug
When you have a TreeView
using ItemsSource
binding and set to SelectionMode="Multiple"
, the IsSelected
property is _not_ changed when clicking on the checkbox. Also, the checkbox is not changed when clicking on the item, either.
Steps to reproduce the bug
Steps to reproduce the behavior:
SelectionMode="Multiple"
to TreeView
IsSelected="{x:Bind IsSelected,Mode=TwoWay}"
to the TreeViewItem
Debug.WriteLine($"Selected changed: {value}");
to the set
statement for IsSelected
in the code-behind file. Include using statement for Debug.Expected behavior
The debug output should show Selected changed: true
when the checkbox is checked and Selected changed: false
when unchecked. Also, the checkbox should be checked/unchecked when the content of the TreeViewItem
is toggled.
Screenshots
Version Info
NuGet package version:
Microsoft.UI.Xaml v2.0.181018003.1
Windows 10 version:
Device form factor:
Additional context
I tried some hacky workarounds to see if I could use Multiple mode, but everytime I changed my IsSelected
property on my viewmodel to True
, the other nodes would immediately all be set to False
Thanks for the report! Our team is on holiday break right now but we'll take a look when we return!
I took a quick look at this and the culprit here seems to be the call to ListView's::PrepareContainerForItemOverride call here. When we data bind a collection to the TreeView (using ItemsSource), set the TreeView to SelectionMode::Multiple and set a (two-way) bound IsSelected property in our bound data collection items, this call to ListView's PrepareContainerForItemOverride() sets the IsSelected property of the bound data item to false
.
This happens because we set the SelectionMode of the ListView to None
in multi-selection mode so there is no concept of "selecting an item" for the ListView. The ListView will thus set the IsSelected property of the ItemContainer to false
which is then reported back to the bound item.
As such, I wrote a quick guard against this call when we are using data binding in SelectionMode::Multiple:
// Don't call ListView's base method when we are using data binding and are in SelectionMode::Multiple
if (!IsContentMode() || !m_isMultiselectEnabled)
{
__super::PrepareContainerForItemOverride(element, item);
}
With additional logic to handle checkbox selection and updating the data-bound IsSelected properties, using IsSelected property binding to select treeview items now works partly. The issue I'm currently facing is this one:
Suppose we have the following data collection we bind to:
private ObservableCollection<TreeViewItemSource> PrepareItemsSource(bool expandRootNode = false)
{
var root0 = new TreeViewItemSource() { Content = "Root.0", IsSelected = true };
var root = new TreeViewItemSource() { Content = "Root", Children = { root0 } };
// TestTreeViewItemsSource
return new ObservableCollection<TreeViewItemSource>{root};
}
We have a root item (Root) with one child (Root.0). The child has its IsSelected property set to true
and we use data binding like this:
<muxcontrols:TreeView
ItemsSource="{x:Bind TestTreeViewItemsSource}"
SelectionMode="Multiple">
<muxcontrols:TreeView.ItemTemplate>
<DataTemplate x:DataType="local:TreeViewItemSource">
<muxcontrols:TreeViewItem
ItemsSource="{x:Bind Children}"
Content="{x:Bind Content}"
IsSelected="{x:Bind IsSelected, Mode=TwoWay}"/>
</DataTemplate>
</muxcontrols:TreeView.ItemTemplate>
</muxcontrols:TreeView>
(Code is a slightly modified version of the MUXControlsTestApp TreeView test code.)
Important here is that root TreeViewItem is not set to be expanded (IsExpanded = false). The expected look is this:
However, the actual behavior is this one:
We first need to actually expand the root item for it to be marked as selected. This is the case because there is no TreeViewItem created for its child (Root.0) yet. A TreeViewItem is only created for the child once we request it to be shown (by expanding its parent). Once it is created, the registered property changed callback for its IsSelected property is called which in turn updates the selection state of its parent and its children (if any). Thus, Root will be set to selected state.
So then, the question I now have...how can we proceed from here? Seems like we currently need the TreeViewItem's children to be able to set the correct selection state on its parent yet we don't have those available when the parent is not in an expanded state initially.
I spent the whole day wrapping my head around what seems to be the same issue as this one until I finally found this issue.
I am also using databinding and the selection state seems to be very unreliable - usually only one checkbox is selected even though multiple should be ticked.
Would be awesome if this could be fixed at some point - looks like the issue is already pretty old. If you need a repro project, let me know and I can make one.
<muxc:TreeView x:Name="treeView" ItemsSource="{x:Bind odFolders}" Expanding="OneDriveFolderView_Expanding"
CanDragItems="False" CanReorderItems="False" SelectionMode="Multiple">
<muxc:TreeView.ItemTemplate>
<DataTemplate x:DataType="local:ODFolder">
<muxc:TreeViewItem ItemsSource="{x:Bind Children, Mode=OneWay}" IsSelected="{x:Bind IsChosen}"
IsExpanded="{x:Bind IsExpanded}" Content="{x:Bind DisplayPath}" Padding="0,0,8,0"
HasUnrealizedChildren="{x:Bind HasUnrealizedChildren, Mode=OneWay}"/>
</DataTemplate>
</muxc:TreeView.ItemTemplate>
</muxc:TreeView>
@Felix-Dev's observation is correct, the rudimentary cause of this problem is the underlying ListView's selection mode being set to none. Skipping the "super" call will cause bunch of other unexpected behaviors. To fix the issue we probably need to either make changes in ListView to make it comply with TreeView or swap out the underlying implementation using ItemsRepeater.
The current workaround is using selection Apis without data binding.
Thanks, that workaround seems to work. I can still use databinding, but have to manually add the item to the SelectedItems
list
Most helpful comment
I took a quick look at this and the culprit here seems to be the call to ListView's::PrepareContainerForItemOverride call here. When we data bind a collection to the TreeView (using ItemsSource), set the TreeView to SelectionMode::Multiple and set a (two-way) bound IsSelected property in our bound data collection items, this call to ListView's PrepareContainerForItemOverride() sets the IsSelected property of the bound data item to
false
.This happens because we set the SelectionMode of the ListView to
None
in multi-selection mode so there is no concept of "selecting an item" for the ListView. The ListView will thus set the IsSelected property of the ItemContainer tofalse
which is then reported back to the bound item.As such, I wrote a quick guard against this call when we are using data binding in SelectionMode::Multiple:
With additional logic to handle checkbox selection and updating the data-bound IsSelected properties, using IsSelected property binding to select treeview items now works partly. The issue I'm currently facing is this one:
Suppose we have the following data collection we bind to:
We have a root item (Root) with one child (Root.0). The child has its IsSelected property set to
true
and we use data binding like this:(Code is a slightly modified version of the MUXControlsTestApp TreeView test code.)
Important here is that root TreeViewItem is not set to be expanded (IsExpanded = false). The expected look is this:

However, the actual behavior is this one:
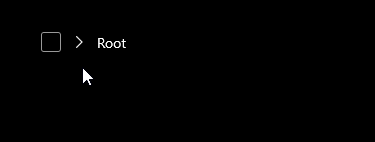
We first need to actually expand the root item for it to be marked as selected. This is the case because there is no TreeViewItem created for its child (Root.0) yet. A TreeViewItem is only created for the child once we request it to be shown (by expanding its parent). Once it is created, the registered property changed callback for its IsSelected property is called which in turn updates the selection state of its parent and its children (if any). Thus, Root will be set to selected state.
So then, the question I now have...how can we proceed from here? Seems like we currently need the TreeViewItem's children to be able to set the correct selection state on its parent yet we don't have those available when the parent is not in an expanded state initially.